Media Queries
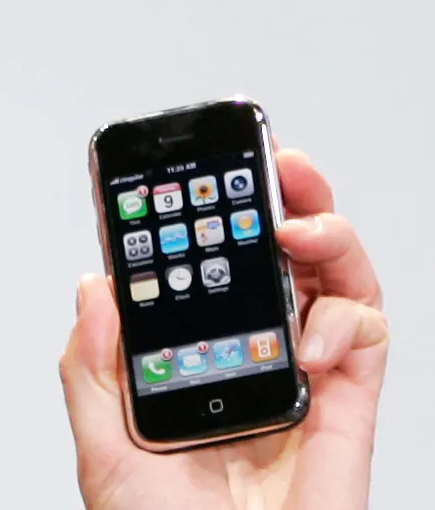
Considering how websites appear across devices is of upmost importance as "users" may encounter them in a variety of conditions. One common way to address the myriad scenarious under which your website may be viewed is through media queries – a CSS feature that enables select styling based on viewing conditions.
Mozilla describes media queries as follows:
Media queries allow you to apply CSS styles depending on a device's general type (such as print vs. screen) or other characteristics such as screen resolution or browser viewport width. Media queries are used for the following:
- To conditionally apply styles with the CSS @media and @import at-rules.
- To target specific media for the
<style>
,<link>
,<source>
, and other HTML elements with the media= attribute.- To test and monitor media states using the Window.matchMedia() and MediaQueryList.addListener() JavaScript methods.
These conditional styles are helpful for changing the appearance of your website depending on the circumstances, such as for a phone, tablet, or if someone were to print your website or view it on a tv!
To use a CSS breakpoint, add the following code to the top of your .html
file:
<meta name="viewport" content="width=device-width, initial-scale=1.0">
This ensures the pixel density is taken into account and your CSS gets read correctly. Then, add the following code at the bottom of your CSS code as an example:
@media only screen and (max-width: 812px) {
// your css goes here
}
this will account for most phones in most orientations. Between the {
and }
brackets you can add CSS that will only be called when the browser window / device width is less than 812px wide. For instance the following code:
body {
background: pink;
}
@media only screen and (max-width: 812px) {
body {
background: beige;
}
}
Will change the background of the body
tag from pink
to beige
when the window is resized below 812px wide. Remember, CSS is read from top to bottom, so the code at the bottom of the page will override that at the top.
You can chain attributes for your CSS together to make it more specific, such as in the following example:
/* Landscape iPhone X */
@media only screen
and (min-device-width: 375px)
and (max-device-width: 812px)
and (-webkit-min-device-pixel-ratio: 3)
and (orientation: landscape) {
// your css goes here
}
which will only target a phone with the screen attributes of an iPhone X (or equivalent model) when in landscape mode.
As mentioned your CSS media queries can extend to the type of "media" on which it is being displayed – such as in print versus on screen. Use the following code to target print:
@media print {
// your css goes here
}
To get familiar with media queries, try the following on your personal site:
- change the background of your body element
- hide and show an element based on a media query
- use screen-resizing as a narrative device
If you want to make an extensive, and highly targeted display for your website, typical breakpoints (as defined by W3Schools) are:
/* Extra large devices (large laptops and desktops, 1200px and up) */
@media only screen and (min-width: 1200px) {...}
/* Large devices (laptops/desktops, 992px and up) */
@media only screen and (min-width: 992px) {...}
/* Medium devices (landscape tablets, 768px and up) */
@media only screen and (min-width: 768px) {...}
/* Small devices (portrait tablets and large phones, 600px and up) */
@media only screen and (min-width: 600px) {...}
/* Extra small devices (phones, 600px and down) */
@media only screen and (max-width: 600px) {...}